Swift Programming Language: A Comprehensive Guide for iOS Application Development
- Abhishek Bagela
- Jan 6
- 3 min read
Swift, introduced by Apple in 2014, has become the cornerstone of iOS application development. Designed for safety, performance, and modern development practices, Swift empowers developers to create robust, high-performance applications. In this blog post, we’ll delve into the Swift programming language, its features, and why mastering Swift is essential for a thriving iOS development career.
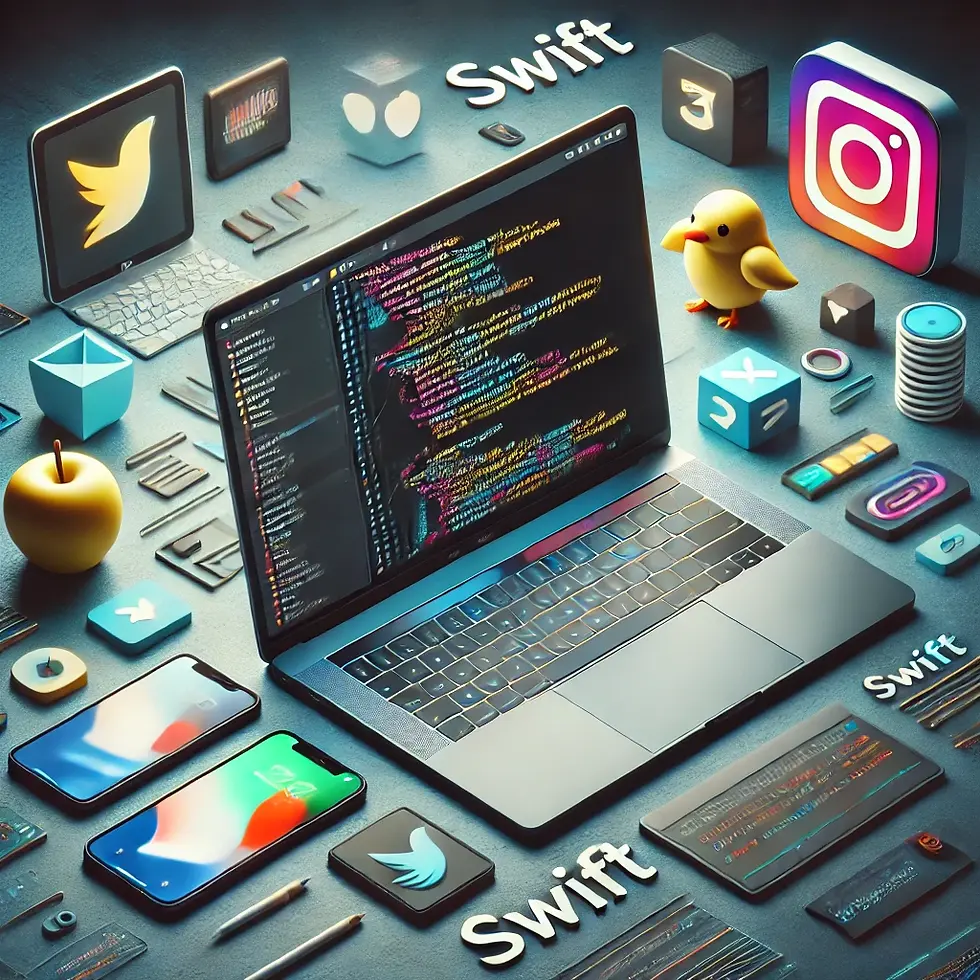
What is Swift?
Swift is a general-purpose, multi-paradigm programming language developed by Apple. It is designed to work seamlessly with Apple’s platforms like iOS, macOS, watchOS, and tvOS. Built as a successor to Objective-C, Swift brings modern features and safety-oriented programming practices to the table.
Key Features of Swift
1. Safety First:
• Swift eliminates common programming errors like null pointer dereferencing using optional types.
• Compile-time checks ensure fewer runtime crashes.
var name: String? = nil // Optional type ensures safe handling of nil values
if let unwrappedName = name {
print("Hello, \(unwrappedName)")
} else {
print("Name is nil")
}
2. Fast and Efficient:
• Swift is built on LLVM (Low-Level Virtual Machine) for high performance.
• Memory management is handled through Automatic Reference Counting (ARC), reducing developer effort.
3. Readable Syntax:
• Swift syntax is concise, clean, and easy to understand, making it beginner-friendly.
let greeting = "Hello, World!"
print(greeting)
4. Interoperability:
• Swift works seamlessly with Objective-C, allowing developers to integrate legacy codebases into new projects.
5. Modern Programming Concepts:
• Supports closures, generics, tuples, and protocols for powerful, reusable code.
func greetUser(name: String) -> String {
return "Hello, \(name)!"
}
print(greetUser(name: "Abhishek"))
Examples and Applications of Swift
1. Building User Interfaces
Swift works seamlessly with SwiftUI, Apple’s declarative UI framework, for creating visually stunning and interactive applications.
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("Welcome to Swift")
.font(.largeTitle)
.padding()
Button(action: {
print("Button clicked!")
}) {
Text("Click Me")
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(8)
}
}
}
}
2. Networking and API Integration
Swift simplifies HTTP requests and API integration using URLSession.
import Foundation
func fetchData(from url: String) {
guard let url = URL(string: url) else { return }
let task = URLSession.shared.dataTask(with: url) { data, response, error in
if let error = error {
print("Error: \(error.localizedDescription)")
return
}
if let data = data {
print("Data received: \(String(data: data, encoding: .utf8) ?? "")")
}
}
task.resume()
}
fetchData(from: "https://jsonplaceholder.typicode.com/posts/1")
3. Game Development
Swift powers game development using frameworks like SpriteKit and SceneKit.
import SpriteKit
class GameScene: SKScene {
override func didMove(to view: SKView) {
let label = SKLabelNode(text: "Hello, Swift!")
label.fontSize = 45
label.fontColor = .white
label.position = CGPoint(x: frame.midX, y: frame.midY)
addChild(label)
}
}
Scope of Swift in iOS Application Development
1. Demand for Swift Developers
• High Demand: iOS applications dominate the mobile app market, driving the need for skilled Swift developers.
• Versatile Applications: Swift is used across various industries, including healthcare, finance, entertainment, and e-commerce.
2. Wide Ecosystem
Swift developers have access to Apple’s rich ecosystem, including Xcode, Swift Playgrounds, and frameworks like Combine, CoreData, and SwiftUI.
3. Career Opportunities
Roles for Swift developers include:
• iOS Developer
• Mobile App Engineer
• Game Developer
• Full-Stack Mobile Developer
According to industry surveys, iOS developers command attractive salaries, often higher than their Android counterparts.
Why Learn Swift?
1. Ease of Learning:
• Swift’s clean syntax makes it accessible to beginners while being powerful for experts.
2. Future-Proof:
• Apple continually invests in Swift, ensuring its longevity and relevance.
3. Community Support:
• A thriving community and extensive documentation make it easy to resolve issues and learn.
4. Open Source:
• Swift is open-source, enabling contributions from developers worldwide and cross-platform usage beyond Apple’s ecosystem.
Conclusion
Swift is not just a programming language—it’s a gateway to building cutting-edge applications for Apple’s ecosystem. Whether you’re a beginner aiming to enter the iOS development world or an experienced developer looking to enhance your skills, mastering Swift is a smart career move. Its modern features, performance, and safety make it indispensable for creating world-class apps.
Start your Swift journey today and unlock limitless opportunities in iOS application development! 🚀
Comments